Swift Algorithmsとは 2020年にAppleが公開したオープンソースパッケージで、順序とコレクションに関する便利なアルゴリズムを提供してくれます。
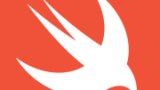
Announcing Swift Algorithms
I’m excited to announce Swift Algorithms, a new open-source package of sequence and collection algorithms, along with their related types.
利用するにはSwift Package Managerで https://github.com/apple/swift-algorithms をプロジェクトに追加し、使用したいファイルにimport Algorithms
を追記してください。
できること
このパッケージにどんなアルゴリズムが含まれているかを簡単に説明していきます。
コンビネーション(Combinations)
コレクションの要素の組み合わせを作ります。数学でやった mCn のアレです。
let numbers = [10, 20, 30, 40]
for combo in numbers.combinations(ofCount: 2) {
print(combo)
}
// [10, 20]
// [10, 30]
// [10, 40]
// [20, 30]
// [20, 40]
// [30, 40]
2つ選択する組み合わせと3つ選択する組み合わせが必要なら以下のように書きます。
let numbers = [10, 20, 30, 40]
for combo in numbers.combinations(ofCount: 2...3) {
print(combo)
}
// [10, 20]
// [10, 30]
// [10, 40]
// [20, 30]
// [20, 40]
// [30, 40]
// [10, 20, 30]
// [10, 20, 40]
// [10, 30, 40]
// [20, 30, 40]
順列(permutations)
コレクションの要素、またはそれらの要素のサブセットの順列を計算する方法です。
let numbers = [10, 20, 30]
for perm in numbers.permutations() {
print(perm)
}
// [10, 20, 30]
// [10, 30, 20]
// [20, 10, 30]
// [20, 30, 10]
// [30, 10, 20]
// [30, 20, 10]
取り出す要素の数を指定したい場合は以下のように書きます。
for perm in numbers.permutations(ofCount: 2) {
print(perm)
}
// [10, 20]
// [10, 30]
// [20, 10]
// [20, 30]
// [30, 10]
// [30, 20]
同じ要素があっても別々のものとして扱われます。
let numbers2 = [20, 10, 10]
for perm in numbers2.permutations() {
print(perm)
}
// [20, 10, 10]
// [20, 10, 10]
// [10, 20, 10]
// [10, 10, 20]
// [10, 20, 10]
// [10, 10, 20]
一意の順列のみを生成するには、uniquePermutations(ofCount:)
メソッドを使用します。
for perm in numbers2.uniquePermutations() {
print(perm)
}
// [20, 10, 10]
// [10, 20, 10]
// [10, 10, 20]
取り出す数に範囲を与えることもできます。
let numbers = [10, 20, 30]
for perm in numbers.permutations(ofCount: 0...) {
print(perm)
}
// []
// [10]
// [20]
// [30]
// [10, 20]
// [10, 30]
// [20, 10]
// [20, 30]
// [30, 10]
// [30, 20]
// [10, 20, 30]
// [10, 30, 20]
// [20, 10, 30]
// [20, 30, 10]
// [30, 10, 20]
// [30, 20, 10]
コメント